-
Notifications
You must be signed in to change notification settings - Fork 0
/
Copy pathEGGERS_CONTENTS.json
1 lines (1 loc) · 20.6 KB
/
EGGERS_CONTENTS.json
1
{"home":{"content":"# Teachback: Intro to JavaScript\n---\nTime to code, fam.\n\n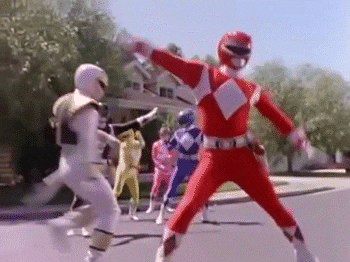\n\n#### Learning Objectives\n---\n1. Understand what JavaScript is and what it is used for\n2. Learn the basic fundamentals of the JavaScript language\n\n#### Rundown\n---\nOk game time. Let's learn some **JavaScript**.\n\n#### [Essential Terminology](#essential-terminology)\nFor your reference.\n\n#### [JavaScript Basics](#javascript-basics)\nFor your reference.\n\n#### [Getting Started](#getting-started)\nFor your reference.\n\n#### [Types of Variables](#types-of-variables)\nThis is where the party begins!\n#### [Functions and Conditionals](#functions-and-conditionals)\n\n","idx":0},"title":{"content":"Javascript Teachback","idx":1},"desc":{"content":"Introduction to JavaScript, y'all","idx":2},"essential-terminology":{"content":"# Essential Terminology\n---\n\n**Learning Objective**: Learn the essential words and concepts that are used on a daily basis by engineers and project/product managers on the job.\n\n---\n\nHere are some words and concepts that will hopefully give you a more holistic view of the more technical aspects of the industry. \n\n#### Define: Program\n---\nDiscrete, highly logical and explicit instructions that are parsed and executed by a computer.\n\nWe call this set of human-readable instructions **source code**, or colloquially, a **computer program**.\n\n**Compilers** can take this source code and transform it into **machine code**, a representation of the source that can be executed by the computer's **central processing unit** or **CPU**. \n\nNot all programs are compiled though, some are **interpreted**. The difference is that compiled languages need a step where the source code is physically transformed into machine code. However, with an interpreted language, this additional step is **excluded** in favor of **parsing** and **executing** the source code directly when the program is run.\n\n#### How programs are written\n---\n\nAll programs are composed with a collection of **fundamental** concepts that, when combined, can essentially dictate a wide variety of tasks a computer can perform.\n\nHere are a collection of these most important concepts:\n\n* **Declarations**: typically, we can store and retrieve data in our programs by associating them with intermediary values that we call **variables**\n* **Expressions**: we use expressions to evaluate stuff. For example, **`2 + 2`** is an example of an expression that will **evaluate** a value, namely 4. \n* **NOTE**: typically we can use expressions and declarations in tandem to perform complex tasks. For instance, we can reference a variable we declared in an expression to help us evaluate new values which can then be stored.\n* **Statements**: statements will use expressions and declarations to alternate a program's **control flow**, which is essentially the order in which declarations, expressions, and other statements are executed.\n\nAside from these fundamental concepts, we also talk a lot about this idea of **algorithms**. An **algorithm** is simple a series of declarations, expressions, and statements that can be used over and over again to solve well defined problems of a certain type.\n\nFor example, we can implement an algorithm that converts temperature from **fahrenheit** to **celsius**. It would look something like this:\n\n1. **Declare** F = 32;\n2. **Expression** ( **F** - 32 ) / 1.8;\n3. **Declare** C = **Evaluated** expression from **[2]**\n\nThis is a form of **pseudo** code where we define the steps a computer program — **any** — computer program can take to convert **fahrenheit** to **celsius**.\n\nThe beauty of programming is that all of it revolves around the same key set of concepts and ideas. For this reason, we do not need to specify any **particular programming language** when discussing the functional aspects of a program.\n\n#### Define: Programming languages\n---\n\nA programming language is a series of **grammar** and **rules** that we can define towards writing source code.\n\nLanguages are effectively different approaches towards communicating the same ideas in programming. Essentially, we can communicate in say both **French** and **English**, what mainly differs is the structure of our sentences and the actual words and sounds themselves.\n\nThe **same analogy** can be made with programming languages.\n\n#### Examples of programming languages\n---\n\nThere are many. Way too many. \n\nHere are some of the most popular ones, though.\n\n1. **JavaScript**: this language is interpreted.\n2. **Python**: this language is interpreted.\n3. **Java**: this language is compiled\n4. **Ruby**: this language is interpreted.\n5. **C/C++**: this language is compiled.\n\nThese languages all build on the same concepts defined above; the main difference lies in **how** they are run (compiled vs interpreted) and also **how** they are used. \n\nIn general, anything programmable can be programmed in each of the languages defined above. However, some languages are better suited for certain tasks above others. \n\nFor example, to perform web programming on the front-end, you'll want to write JavaScript. This is because all browsers collectively support running javascript within it's environment. \n\n","idx":3},"javascript-basics":{"content":"# JavaScript Basics\n---\n\nLet's begin by defining what javascript actually is, how we can load javascript into our webpages, and how we can write a few lines of super basic javascript syntax.\n\n## [JS Global Notes](http://samantha.fewd.us/#fork/mottaquikarim/FEWD627_JS_Basics) | [LIVE Instructor View](http://samantha.fewd.us/#broadcast/mottaquikarim/FEWD627_JS_Basics)\n\n#### Learning Objectives\n---\n1. What can we do with javascript code?\n2. How can we load javascript code into our browser?\n3. What are the different ways we can store, retrieve, and use data in javascript?\n\n#### What is JavaScript\n---\nOriginally called **Mocha**, then **LiveScript**, then renamed to **JavaScript**.\n\n2. It's a subclass of ECMAScript, a standardization maintained by Ecma International\n3. Basically, Ecma is the **Webster's Dictionary** of JavaScript\n\n### What can you do with Javascript?\n\nJavaScript runs in the <strong>browser</strong>, meaning it is used heavily in user-interaction.\nIn other words, with javascript, you can...\n\n#### Animate stuff\n---\nYou can use javascript to create for example an infinite random prop loop.\n\n<iframe scrolling='no' src='//codepen.io/mottaquikarim/embed/jgIbd/?height=268&theme-id=820&default-tab=result' frameborder='no' allowtransparency='true' allowfullscreen='true' style='min-height: 268px !important;'>See the Pen <a href='http://codepen.io/mottaquikarim/pen/jgIbd/'>jQuery Random Height/Width</a> by Mottaqui Karim (<a href='http://codepen.io/mottaquikarim'>@mottaquikarim</a>) on <a href='http://codepen.io'>CodePen</a>.\n</iframe>\n\n#### Allow the user to control stuff\n---\nYou can allow the user to take control of a UI element and dictate its state.\n\n<iframe height=\"300\" src=\"//jsfiddle.net/2jwnjwfd/1/embedded/result\" allowfullscreen=\"allowfullscreen\" frameborder=\"0\"></iframe>\n\n#### Mess with people's minds\n---\nThis is just a pretty cool masking example that is actually pretty hard to pull off with web dev tech (but can be done, as shown here thanks to javascript).\n\n(**FYI**: this formed the basis of [this website](http://maveron.com/) that I built back in the day).\n\n<iframe scrolling='no' src='//codepen.io/mottaquikarim/embed/DABIG/?height=268&theme-id=820&default-tab=result' frameborder='no' allowtransparency='true' allowfullscreen='true' style='min-height: 268px !important;'>See the Pen <a href='http://codepen.io/mottaquikarim/pen/DABIG/'>Mask Effect demo</a> by Mottaqui Karim (<a href='http://codepen.io/mottaquikarim'>@mottaquikarim</a>) on <a href='http://codepen.io'>CodePen</a>.\n</iframe>\n\n#### Determine the state of something on a page in real time\n---\nWe are using something called conditionals here.\n\n<iframe width=\"100%\" height=\"500\" style=\"height: 400px;\" src=\"//jsfiddle.net/hrfq4qrj/1/embedded/result\" allowfullscreen=\"allowfullscreen\" frameborder=\"0\"></iframe>\n\n#### Load in data dynamically (this is the best one)\n---\nBasically, we can interact with API data without ever reloading the page!","idx":4},"getting-started":{"content":"# Getting Started\n---\n\nBefore we begin, let us do some basic bookkeeping.\n\n## [JS Global Notes](http://samantha.fewd.us/#fork/mottaquikarim/FEWD627_JS_Basics) | [LIVE Instructor View](http://samantha.fewd.us/#broadcast/mottaquikarim/FEWD627_JS_Basics)\n\n#### Exercise\n---\n\nCreate a new project folder with a **script** tag and include a **main.js** file. Verify that you linked this file properly.\n\n**Question**: How would you know that your **main.js** file is loading without knowing any actual lines of javascript?!\n\n#### Linking to Javascript\n---\n\n**How do we set up javascript to run in the browser?**\n\n1. The precedent is similar to what we do to link CSS files — we employ a tag to tell the HTML page where the javascript code for that page lives.\n\n2. However, we do NOT use the link tag...\n\n3. Instead, we do the following:\n\n```html\n<!doctype html>\n<html>\n\t<head>\n\t\t<!-- Do NOT link to your javascript here -->\n\t</head>\n\t<body>\n\n\t\t<script src=\"PATH_TO_YOUR_JS_FILE\"></script>\n\t</body>\n</html>\n```\n\n**NOTE the `<script>` tag**\n\n1. This is a new tag we have never seen before; remember that the `<link>` tag is for CSS files and the `<script>` tag is for javascript files (for now).\n2. the `src` attribute is what we use to link to the external js file\n3. remember to CLOSE your script tag, unlike the `<link>` tag, `<script>` is NOT self closing!\n\n**Commenting + ( basic debugging of ) code in JavaScript**\n\n```js\n\n// this is an inline comment\n\nconsole.log('You can write debugging statements like so');\n\n/*\n\tthis\n\t\tis\n\t\t\ta\n\t\t\t\tmultilined\n\t\t\t\t\t\tcomment\n*/\n```\n\n","idx":5},"types-of-variables":{"content":"# Types of Variables\n---\n\n## [JS Global Notes](http://samantha.fewd.us/#fork/mottaquikarim/FEWD627_JS_Basics) | [LIVE Instructor View](http://samantha.fewd.us/#broadcast/mottaquikarim/FEWD627_JS_Basics)\n\n#### Exercise\n---\n\nWe want to learn enough javascript to be able to solve the following PSET.\n\n#### [Basic Vars Review](http://samantha.fewd.us/#fork/mottaquikarim/Basic_Vars_Review) | [LIVE Instructor View](http://samantha.fewd.us/#broadcast/mottaquikarim/Basic_Vars_Review)\n\nThis will help you get your fingers warm re: simple javascript variable declarations.\n\n\n#### Definitions\n---\n\nA variable type is a way to classify the different kinds of data we can save to a variable. There are exactly 6 types of variables:\n\n#### Primitives\n\n* `undefined`\n* `null`\n* boolean\n* number\n* string\n\n#### Non Primitive\n\n* Object\n\n### Primitives\n\nA Primitive type is a most basic bit of information that you can store. For example, a number is a primitive because it cannot be made up of any of the other types of variables\n\n<strong>Alternate definition</strong>: Think of this as an atom -- atoms are atoms because we cannot break them down into any more basic bits, same goes for primitives\n\n#### `undefined`\n\nUndefined is the default state of any variable. Basically means the variable is empty or has not yet been assigned a value, primitive or otherwise\n\n#### `null`\n\nThe null variable is different from the `undefined` type, but only subtly so.\n\n1. the `null` type is assigned to a variable, but its \"value\" is empty.\n2. the `undefined` type is by default the value of each variable that is declared but not defined\n3. #esoteric\n\n**[Example](http://fewd.us/howdoi/#/problem/5131)**\n\n#### Booleans\n\nTrue or false. Basically.\n\n```javascript\nvar myBooleanValue = true; // true\nvar myBooleanValueThatIsFalse = false; // false\nconsole.log( typeof myBooleanValue );\n```\n\n#### Numbers\n\nNumeric values that can be operated upon via the standard rules of arithmetic.\n\n```javascript\nvar myNumber = 1;\nvar pi = 3.14159; // ...approximately\n```\n\n\n#### Strings\n\nThis one is interesting, we use this to represent text. Anything between the quotations (double or single, doesn't matter as long as you are consistent) is treated as a number.\n\nSo...\n\n```javascript\nvar myName = 'Taq Karim';\nvar dudeThisIsAString = '105';\n\nvar doubleQuotesRCool2 = \"Look ma! I'm double quoted\";\n```\n\n### Non-Primitives or: how I learned to stop worrying and Love the Object.\n\nObjects are the bees-knees yo.\n\n<div>Seriously.</div>\n\n<strong>Objects are a collection of properties where each property is a primitive type</strong>.\n\n#### In other words...\n```javascript\n// just primitives\nvar someNumber = 1;\nvar someBool = false;\nvar someNullItem = null;\n\n\n// as an object...\nvar myObject = {\n\tsomeNumber: 1\n\t, someBool: false\n\t, someNullItem: null\n}; // notice the use of \":\" instead of \"=\"\n\n// to access these items:\nconsole.log( myObject.someNumber );\nconsole.log( myObject.someBool );\n```","idx":6},"functions-and-conditionals":{"content":"# Functions and Conditionals\n---\nLet's learn to actually do stuff with javascript now. Functions and conditionals well help us automate programming tasks and also build in decision making capabilities into our programs.\n\n## [JS Global Notes](http://samantha.fewd.us/#fork/mottaquikarim/FEWD627_JS_Basics) | [LIVE Instructor View](http://samantha.fewd.us/#broadcast/mottaquikarim/FEWD627_JS_Basics)\n#### Learning Objectives\n---\n1. Understand how to use functions to abstract away common coding tasks\n2. Use conditionals to build in decision making into our code.\n\n#### Exercises\n---\nThe following PSET contains 10 problems. \n#### [PSET - Functions](http://samantha.fewd.us/#fork/mottaquikarim/Functions_Practice) | [LIVE Instructor Notes](http://samantha.fewd.us/#broadcast/mottaquikarim/Functions_Practice)\nWe will attempt to solve some or MOST of them.\n\n#### [PSET - Conditionals](http://samantha.fewd.us/#fork/mottaquikarim/Conditionals) | [LIVE Instructor Notes](http://samantha.fewd.us/#broadcast/mottaquikarim/Conditionals)\nTry to solve all of these\n\n#### [Problem - Calculate Grade](http://samantha.fewd.us/#fork/mottaquikarim/Calculate_Grade) | [LIVE Instructor Notes](http://samantha.fewd.us/#broadcast/mottaquikarim/Calculate_Grade)\nThis one is more difficult, try it out though!\n\n#### Functions\n---\n\nFunctions are essentially actions. We use functions to save a set of `instructions` that we can tell javascript to use over and over again. Functions have names, just like variables, but they hold more than just primitive values.\n\n#### Example\n\nThis is a function declaration.\n\n```javascript\nfunction doSomething() {\n\talert('doing something!');\n}\n```\n\nHere is how we would call that function\n```javascript\n// copying over last code snippet for convenience\nfunction doSomething() {\n\talert('doing something!');\n}\n\n// calling it now\ndoSomething();\n```\n\n#### Alternate way to do this\n\n```javascript\nvar doSomething = function() {\n\talert('doing something!');\n}\n\n// call it\ndoSomething();\n```\n\n#### Conditionals\n---\n\nUsed do perform logic\n\n### Rundown\n<a>JavaScript Logical Operators</a>\n<ul>\n <li>`===`</li>\n <li>`!==`</li>\n <li>`>`</li>\n <li>`<`</li>\n <li>`>=`</li>\n <li>`<=`</li>\n <li>`&&`</li>\n <li>`||`</li>\n <li>`!`</li>\n</ul>\n```js\n// Comparison operators\n// you can evaluate a situation by comparing one value in the script\n// to what you expect it might be\n// the result will be a boolean: true or false\n\n/*\n * the strict equality checking operator\n * SYMBOL: ===\n */\nvar three = 3;\nvar equalityCheck = ( three === 3 );\nconsole.log( 'three === 3', equalityCheck );\n// note that equalityCheck, and ALL OTHER COMPARISON OPERATORS\n// evaluate to booleans\nconsole.log( 'typeof equalityCheck', typeof equalityCheck );\n\nvar equalityCheck2 = ( three === '3' ); // false because three is Number\n // but '3' is a string\nconsole.log( \"( three === '3' )\", equalityCheck2 );\n// ALWAYS USE THE STRICT EQUALITY OPERATOR!!\n\n/*\n * the strict NOT EQUAL checking operator\n * SYMBOL: !==\n */\n\nvar notEqualityCheck = ( three !== 4 ); // should be true\nvar notEqualityCheck2 = ( three !== 3 ); // should be false;\n\nconsole.log( '( three !== 4 )', notEqualityCheck );\nconsole.log( '( three !== 3 )', notEqualityCheck2 );\n\n/*\n * the greater than operator\n * SYMBOL: >\n */\nvar greaterThan = ( three > 4 ); // returns false\nvar greaterThan2 = ( three > 2 ); // true\nconsole.log( '( three > 4 )', greaterThan );\nconsole.log( '( three > 2 )', greaterThan2 );\n\n/*\n * the less than operator\n * SYMBOL: <\n */\nvar lessThan = ( three < 2 ); // returns false\nvar lessThan2 = ( three < 5 ); // true\nconsole.log( '(three < 2)', lessThan );\nconsole.log( '(three < 5)', lessThan2 );\n \n/*\n * the greater than or equal to operator\n * SYMBOL: >=\n */ \nvar greaterThanOrEqualTo = ( three >= 4 ); // false\nvar greaterThanOrEqualTo2 = ( three >= 3 ); // true\nconsole.log( '( three >= 4 )', greaterThanOrEqualTo );\nconsole.log( '( three >= 3 )', greaterThanOrEqualTo2 );\n\n/*\n * the less than or equal to operator\n * SYMBOL: <=\n */ \nvar lessThanOrEqualTo = ( three <= 2 ); // false\nvar lessThanOrEqualTo2 = ( three <= 3 ); // true\nconsole.log( '( three <= 2 )', lessThanOrEqualTo );\nconsole.log( '( three <= 3 )', lessThanOrEqualTo2 );\n\n/*\n * LOGICAL OPERATORS\n * logical operators allow you to compare the results of more than one\n * comparison operator\n * TYPES: '&&', '||'\n */ \n\n/*\n * the AND operator\n * SYMBOL: &&\n * returns true only when both expressions evaluate to true\n */ \nvar andOp = ((2 < 5) && (3 >=2)); // is 2 less than five AND\n // is 3 greater than or equal to 2\nconsole.log( '((2 < 5) && (3 >=2))', andOp );\nconsole.log( 'typeof andOp', andOp );\n// NOTE, we can string together as many ANDs as we need...\n\n/*\n * the OR operator\n * SYMBOL: ||\n * returns true when ONE of the expressions are true\n */ \nvar orOp = ((2 < 5) || (2 < 1)); // if EITHER one of these exprs are true\n // then will evaluate to true\nconsole.log( '((2 < 5) || (2 < 1))', orOp );\n// NOTE, we can string together as many ORs as we need...\n\n/*\n * the NOT operator\n * SYMBOL: !\n * returns opposite of evaluated expression\n */ \nvar notOp = !(2 < 1);\nconsole.log( '!(2 < 1)', notOp );\n\n// Complete example\n\nfunction checkNumber () {\n \n var n = prompt(\"Enter a number\", \"5\")\n , entered = \"You entered a number between\"; \n\n if (n >= 1 && n < 10) {\n alert(entered + \" 0 and 10\");\n }\n else if (n >= 10 && n < 20) {\n alert(entered + \" 9 and 20\");\n }\n else if (n >= 20 && n < 30) { \n alert(entered + \" 19 and 30\");\n }\n else if (n >= 30 && n < 40) {\n alert(entered + \" 29 and 40\");\n }\n else if (n >= 40 && n <= 100) {\n alert(entered + \" 39 and 100\");\n }\n else if (n < 1 || n > 100) {\n alert(\"You entered a number less than 1 or greater than 100\");\n }\n else {\n alert(\"You did not enter a number!\");\n }\n}\n\n\n```\n\n<a>The conditional block</a>\n<ul>\n <li>the `if` statement</li>\n <li>the `if/else` statement</li>\n <li>the `if/else if/else` statement</li>\n</ul>\n```js\n// the if..else statement check a condition\n// if it resolves to true the first code block is run\n// if the condition resolved to false the second code block is run instead\n\nvar pass = 50 // pass mark\n , score = 75 // current score\n , msg; // message\n \n// select message to write based on score \nif ( score >= pass ) { \n // conditional statement\n // if code block\n // anything in here will be run if\n // the stuff in the (...) evaluates to true\n msg = \"Congratulations you passed!\"; \n} \nelse { // else code block; if the obove is NOT true then...\n msg = \" Try again :( \"; \n}\n// note this example of how we can use the if/else\n// to set the value of a variable\nconsole.log( msg ); \n\n/*\n * the if/else if/else block\n */\n\nvar passingGrade;\nif ( score < 50 ) {\n passingGrade = \"F\";\n}\nelse if ( score < 60 ) {\n passingGrade = \"D\";\n}\nelse if ( score < 70 ) {\n passingGrade = \"C\";\n}\nelse if ( score < 80 ) {\n passingGrade = \"B\";\n}\nelse if ( score < 90 ) {\n passingGrade = \"A\"\n}\nelse {\n passingGrade = \"A+\";\n}\n\nconsole.log( \"Your grade is: \", passingGrade );\n\n\n\n\n\n \n \n \n\n\n\n\n\n\n\n```","idx":7},"__list__":["home","title","desc","essential-terminology","javascript-basics","getting-started","types-of-variables","functions-and-conditionals"]}